首先,确保你的Python环境中已安装telnetlib库。如果未安装,可以通过以下命令进行安装:
```bash pip install telnetlib ```
接下来,编写Python脚本。以下是一个示例脚本,展示了如何批量登录华为交换机并执行一些基本命令:
```python import telnetlib
def telnet_login(host, username, password, commands): try: 连接Telnet服务器 tn = telnetlib.Telnet(host, timeout=10) 读取登录提示并输入用户名 tn.read_until(b"Username:") tn.write(username.encode('ascii') + b"\n") 读取密码提示并输入密码 tn.read_until(b"Password:") tn.write(password.encode('ascii') + b"\n") 登录成功后执行命令 for cmd in commands: tn.write(cmd.encode('ascii') + b"\n") output = tn.read_until(b">").decode('ascii') 根据设备的提示符调整 print(output) 打印命令输出 退出Telnet tn.write(b"quit\n") tn.close() except Exception as e: print(f"Telnet connection to {host} failed: {e}")
if __name__ == "__main__": host_list = ["192.168.56.2"] 设备的ip地址列表 username = "huawei" password = "huawei" commands_to_run = ["display version", "display interface brief"] 要执行的命令列表 for host in host_list: print(f"Connecting to {host}...") telnet_login(host, username, password, commands_to_run) ```
在这个脚本中:
1. `telnet_login`函数负责建立Telnet连接,输入用户名和密码,并执行指定的命令。 2. `host_list`是要连接的设备IP地址列表。 3. `username`和`password`是用于Telnet认证的用户名和密码。 4. `commands_to_run`是在每台设备上要执行的命令列表。
脚本会遍历设备列表,对每个设备进行Telnet登录并执行指定的命令,最后输出命令的结果。
为了确保脚本能够正常运行,需要根据实际环境调整一些参数,例如设备的IP地址、用户名、密码以及设备的提示符。此外,确保网络防火墙允许Telnet通信。
通过这种方式,可以高效地批量管理华为交换机,节省时间和精力。需要注意的是,Telnet通信是明文传输,存在安全风险,因此在生产环境中建议使用更安全的SSH协议进行设备管理。
总之,利用Python和telnetlib库,可以轻松实现华为交换机的批量登录和管理,提升网络管理的自动化水平。
如何使用python通过telnet批量登录华为交换机,下图是演示环境
配置telnet服务器
这里我们在R1路由器上配置下telnet登录配置,从而能够通过本地telent登录;
[R1]telnet server enable [R1]user-interface vty 0 4 [R1-ui-vty0-4]authentication-mode aaa [R1]aaa [R1-aaa]local-user huawei password cipher huawei [R1-aaa]local-user huawei service-type telnet [R1-aaa]local-user huawei privilege level 3
在本地验证下,可以登录路由器R1
使用python脚本登录设备
要通过 Python 脚本使用 Telnet批量登录华为交换机,你可以使用第三方库如 telnetlib 来实现。
首先,确保你已经安装了 telnetlib 库,如果没有安装,可以使用以下命令安装:
pip install telnetlib
然后,可以使用下面的 Python 脚本来实现批量 Telnet 登录和执行命令:
import telnetlib def telnet_login(host, username, password, commands): try: 连接 Telnet 服务器 tn = telnetlib.Telnet(host) 输入用户名 tn.read_until(b"Username:") tn.write(username.encode('ascii') + b"\n") 输入密码 tn.read_until(b"Password:") tn.write(password.encode('ascii') + b"\n") 登录成功后执行命令 for cmd in commands: tn.write(cmd.encode('ascii') + b"\n") output = tn.read_until(b">").decode('ascii') 根据设备的提示符来调整 print(output) 打印命令输出 print("1") 退出 Telnet tn.write(b"quit\n") tn.close() except Exception as e: print(f"Telnet connection to {host} failed: {e}") if __name__ == "__main__": host_list = ["192.168.56.2"] 设备的 IP 地址列表 username = "huawei" password = "huawei" commands_to_run = ["display version", "display interface brief",""] 要执行的命令列表 for host in host_list: print(f" Connecting to {host}...") telnet_login(host, username, password, commands_to_run)
在这个脚本中:
- telnet_login 函数用于连接到 Telnet 服务器,并执行指定的命令。
- host_list 是要连接的设备列表。
- username 和 password 是用于 Telnet 认证的凭据。
- commands_to_run 是要在每台设备上执行的命令列表。
- 脚本会遍历设备列表,对每个设备执行 Telnet 登录和指定的命令,并输出命令的结果。
python执行结果
Connecting to 192.168.56.2... Info: The max number of VTY users is 10, and the number of current VTY users on line is 1. The current login time is 2024-04-09 22:21:33. display version Huawei Versatile routing Platform Software VRP (R) software, Version 5.110 (eNSP V100R001C00) Copyright (c) 2000-2011 HUAWEI TECH CO., LTD display interface brief PHY: Physical *down: administratively down ^down: standby (l): loopback (s): spoofing (b): BFD down (e): ETHOAM down (d): Dampening Suppressed InUti/OutUti: input utility/output utility Interface PHY Protocol InUti OutUti inErrors outErrors Ethernet0/0/0 up up 0% 0% 0 0 Ethernet0/0/1 up up 0% 0% 0 0 GigabitEthernet0/0/0 down down 0% 0% 0 0 GigabitEthernet0/0/1 down down 0% 0% 0 0 GigabitEthernet0/0/2 down down 0% 0% 0 0 GigabitEthernet0/0/3 down down 0% 0% 0 0 NULL0 up up(s) 0% 0% 0 0 Serial0/0/0 down down 0% 0% 0 0 Serial0/0/1 down down 0% 0% 0 0 Serial0/0/2 down down 0% 0% 0 0 Serial0/0/3 down down 0% 0% 0 0 ---END---
本站声明:网站内容来源于网络,如有侵权,请联系我们,我们将及时处理。
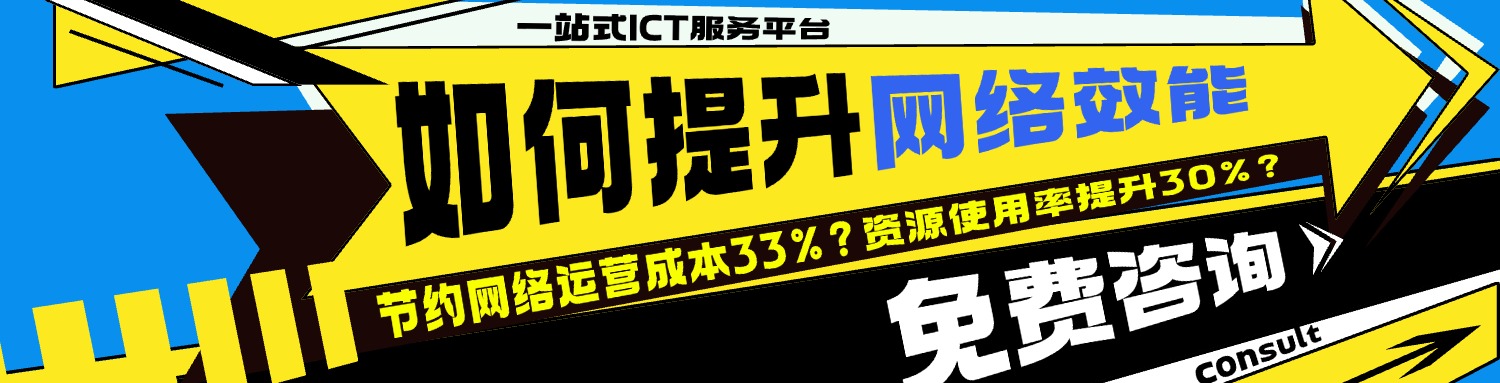